Notice
Recent Posts
Recent Comments
Link
250x250
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- 파이썬 for
- 리액트 훅
- 파이썬 slice
- 프로그래머스
- 리액트 공식문서
- REACT
- 자바스크립트
- 타입스크립트 리액트
- useEffect
- 파이썬 replace
- 내일배움캠프 프로젝트
- typeScript
- 파이썬 for in
- 리액트 공식 문서
- 한글 공부 사이트
- 파이썬 반복문
- 코딩테스트
- 리액트 프로젝트
- Next 팀 프로젝트
- 내배캠 프로젝트
- tanstack query
- JavaScript
- 타입스크립트
- React Hooks
- 리액트
- useState
- 파이썬 딕셔너리
- 내일배움캠프 최종 프로젝트
- 파이썬 enumerate
- 내일배움캠프
Archives
- Today
- Total
sohyeon kim
[React] axios.post/delete/patch : HTTP 통신으로 데이터 생성/삭제/수정하기 본문
728x90
반응형
2024.02.19 - [React] - [React] axios.get : HTTP 통신으로 데이터 가져오기
[React] axios.get : HTTP 통신으로 데이터 가져오기
💡 Axios : Promise 를 기반으로 해 HTTP 통신을 할 수 있는 라이브러리 먼저 npm install axios or yarn add axios ~ npm install json-server or yarn add json-server ~ 혹시나 EACCES 에러가 뜬다면 sudo npm install json-server 루트
aotoyae.tistory.com
💡 Axios : Promise 를 기반으로 해 HTTP 통신을 할 수 있는 라이브러리
💡 POST : input 에 값을 받고 데이터를 생성해 보자!
App.jsx
import axios from "axios";
import { useEffect, useState } from "react";
function App() {
const [todos, setTodos] = useState(null);
const [inputValue, setInputValue] = useState({ // input 값 저장
title: "", // 객체 형태로 넘겨야 해서 이런 식으로 씀
});
const fetchTodos = async () => {
const { data } = await axios.get("http://localhost:4000/todos");
setTodos(data);
};
const onSubmitHandler = async () => {
axios.post("http://localhost:4000/todos", inputValue);
setTodos([...todos, inputValue]); // 리렌더링 되도록 다시 셋
};
useEffect(() => {
fetchTodos();
}, []);
return (
<div>
<div>
<form
onSubmit={(e) => {
e.preventDefault(); <!-- submit 은 새로고침을 일으키니 막아두기 -->
onSubmitHandler();
}}
>
<input
type="text"
value={inputValue.title}
onChange={(e) => setInputValue({ title: e.target.value })}
<!-- 객체라서 요렇게 써야 함. 타이틀만 바꿔줄꺼니까 -->
/>
<button type="submit">추가</button>
</form>
</div>
<div>
{todos?.map((todo) => (
<div key={todo.id}>
{todo.id} : {todo.title}
</div>
))}
</div>
</div>
);
}
export default App;
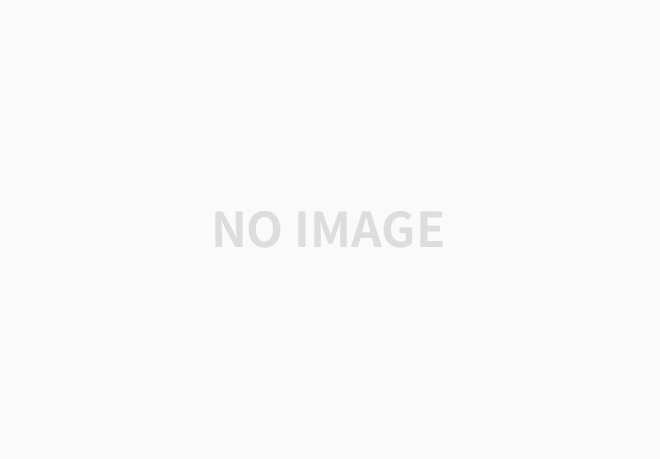
title 값만 들어가 오류가 뜨는데, 우선 새로고침을 하면 id 값이 자동생성되어 들어가있다.
➡️ db 에 id 가 자동으로 들어가고 있지만, state 는 id 를 알 수 없기 때문
그러니 fetchTodo() 로 데이터를 아예 다시 불러온다.
😲 아래와 같이 변경한다.
const onSubmitHandler = async () => {
axios.post("http://localhost:4000/todos", inputValue);
fetchTodos();
};
💡 DELETE : 삭제 기능을 만들어보자!
todo 마다 삭제버튼을 만들어주고, 삭제 함수를 연결한다.
const onDeleteBtnHandler = async (id) => {
axios.delete(`http://localhost:4000/todos/${id}`); // 삭제할 todo 의 id 를 보낸다.
setTodos(todos.filter((todo) => todo.id !== id)); // 리렌더링 하기 위해 필터링
};
// ...
return (
// ...
{todos?.map((todo) => (
<div key={todo.id}>
{todo.id} : {todo.title}
<button onClick={() => onDeleteBtnHandler(todo.id)}>삭제</button>
</div>
))}
)
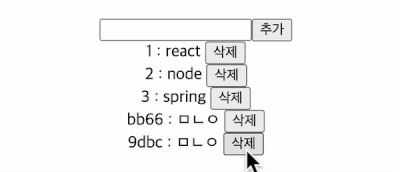
💡 PATCH : 데이터를 수정해보자!
form 태그 위에 새로운 div 영역을 만들자.
수정할 todo 의 id 와 수정할 내용을 저장하기 위해 새 useState 를 만든다.
함수 만들어서 실행
const [targetId, setTargetId] = useState("");
const [content, setContent] = useState("");
// ...
const onUpdateBtnHandler = async () => {
axios.patch(`http://localhost:4000/todos/${targetId}`, { // 수정할 todo의 아이디와
title: content, // 내용을 보낸다.
});
setTodos(
todos.map((todo) => { // todos 를 맵으로 돌려 id 가 같은 todo 의 내용을 바꾼다.
return todo.id == targetId ? { ...todo, title: content } : todo; // id 들의 형이 달라 동등연산자 사용
})
);
};
// ...
return (
// ...
<div>
<input
type="text"
placeholder="수정할 아이디"
value={targetId}
onChange={(e) => setTargetId(e.target.value)}
/>
<input
type="text"
placeholder="수정할 내용"
value={content}
onChange={(e) => setContent(e.target.value)}
/>
<button onClick={onUpdateBtnHandler}>수정</button>
</div>
// ...
)
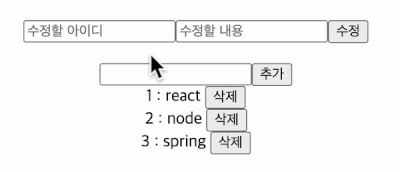
728x90
반응형
'React' 카테고리의 다른 글
[React] env : npm, vite 환경 변수 사용하기, 변수 숨기기 (0) | 2024.02.19 |
---|---|
[React] Fetch & Axios 의 차이 (0) | 2024.02.19 |
[React] axios.get : HTTP 통신으로 데이터 가져오기 (0) | 2024.02.19 |
[React] json-server : 간단한 DB & API 서버 생성 패키지 (0) | 2024.02.16 |
[React] Redux toolkit : store 만들기 (0) | 2024.02.16 |